To add a background is easy as you already know for sure. Just add another layer in the main timeline and draw your background (I drew mine in Freehand and pasted it into Flash); is good to name the different layers so you know where the things are.
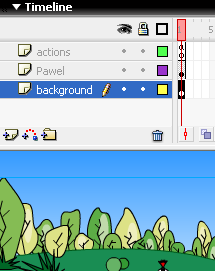
The arrow can be drawn in Flash as well (I used Photoshop to draw mine). The arrow should be a movieclip and we should leave it in the library, not on the stage.
Open the library and right click the arrow movieclip so you can open the Linkage Properties panel, if you tick in linkage options Export for Actionscript by default the identifier will be arrow, so leave it like that.
We will add to the script a couple of variables, one to declare the speed of our arrow and another to allow us to shoot one arrow at a time and we need a function to update our arrow.
Now the script look like:
//---- variables ----If we test our movie, there are a couple of weird things going on.
var steps:Number = 5;
var spriteX:Number = 265;
var spriteY:Number = 265;
var speed:Number = 25;
var arrowActive:Boolean = false;
//---- functions ----
function checkKeys() {
if (Key.isDown(Key.RIGHT) && spriteX<510) {
spriteX -= steps;
knight.legs.play();
}
if (Key.isDown(Key.UP) && arrowActive == false) {
knight.arms.play();
attachMovie("arrow", "arrows", 8);
arrows._x = spriteX;
arrows._y = spriteY+50;
arrowActive = true
}
}
function updatePawel() {
knight._x = spriteX;
knight._y = spriteY;
}
function updateArrow() {
if (arrowActive == true) {
arrows._y -= speed;
}
if (arrows._y<-10) { arrowActive = false; removeMovieClip(arrows); } } this.onEnterFrame = function() { checkKeys(); updatePawel(); updateArrow(); };
The first thing is, the arrows are coming from the back of Pawel, so there is a problem with the "depths". And the second problem is that the arrow is active and moving before the animation of the arms is running!
Let's see first the new things in our script and then let's fix the problems.
var speed:Number = 25; var arrowActive:Boolean = false;
As said before, speed is the number of pixels our arrow will move and in arrowActive we are declaring the boolean value of this variable, as is false means that the arrow has not been shot.
if (Key.isDown(Key.UP) && arrowActive == false) { knight.arms.play(); attachMovie("arrow", "arrows", 8); arrows._x = spriteX; arrows._y = spriteY+50; arrowActive = true }
We have added another conditional, arrowActive. If it is false, the knight will move the arms, an instance of the movieclip arrow will be attached, the name of this new instance is arrows and the depth (how close to you is this object related to the other objects on the stage) is 8, so is on top of every object with a lower depth.
That's why our hero seems to be behind the arrow!
By default, the depth is 0 (zero).
function updateArrow() { if (arrowActive == true) { arrows._y -= speed; } if (arrows._y<-10) { arrowActive = false; removeMovieClip(arrows); } }
This function is telling flash that if the arrow is active, the arrow will have the speed before declared (25) and if the arrow's Y position is lower than -10 (outside of the stage), the arrow will not be active and the instance of the movieclip arrow will be removed.
this.onEnterFrame = function() { checkKeys(); updatePawel(); updateArrow(); };
In real time, flash will be checking the situation of the arrows.
Now let's fix the bugs.
We need to change the depth of our hero adding this line to our script:
knight.swapDepths(10);
And to activate the arrow in the right time, we will edit our arms movieclip.
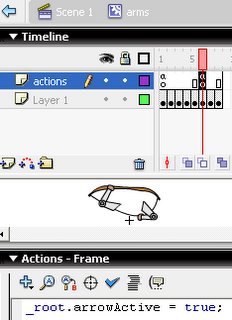
When the hero is about to shoot the arrow (in the animation) we added the following script:
_root.arrowActive = true;
Where _root is the main timeline, so this code aplies to the variable declared in the main timeline and is activating the arrow.
So far, the latest version of the script is:
//---- variables ----As we are activating the arrows in the arms animation, we are leaving //arrowActive = true as a comment only (everything written after "//" in the same line is a comment).
var steps:Number = 5;
var spriteX:Number = 265;
var spriteY:Number = 265;
var speed:Number = 25;
var arrowActive:Boolean = false;
//---- properties ----
knight.swapDepths(10);
//---- functions ----
function checkKeys() {
if (Key.isDown(Key.RIGHT) && spriteX<510)>40) {
spriteX -= steps;
knight.legs.play();
}
if (Key.isDown(Key.UP)&& arrowActive == false) {
knight.arms.play();
attachMovie("arrow", "arrows", 8);
arrows._x = spriteX;
arrows._y = spriteY+50;
//arrowActive = true
}
}
function updatePawel() {
knight._x = spriteX;
knight._y = spriteY;
}
function updateArrow() {
if (arrowActive == true) {
arrows._y -= speed;
}
if (arrows._y<-10) { arrowActive = false; removeMovieClip(arrows); } } this.onEnterFrame = function() { checkKeys(); updatePawel(); updateArrow(); };
Watch the sample here.
Next part of this tutorial, coming soon.
No comments:
Post a Comment