We need the dragons.
Again, as we did with the arrow, background, etc. we need to draw our dragons, I drew mine first using Freehand and then pasted into flash.
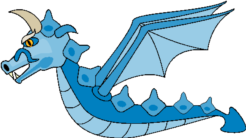
At the moment it will be a still image, although we can make a little animation of its wings.
We don't need instances of our dragon on the stage but we need it in the library as a movieclip.
Open the library and right click on the dragons movieclip to open the linkage properties window, tick Export for Actionscript and leave the identifier as default (dragon).
Now the actionscript.
The first three new things to be added are the variables:
var dragons:Number = 3;
var i:Number = 0;
var score:Number = 0;
dragons is the number of dragons we will have on the stage.
The variable i is a bit difficult to explain as is going to help us in more than one thing, i.e. to give a specific name to each dragon.
score is to store our score, we can add a dinamic text box on the stage with variable name score so we now how good we are killing dragons.
We need to add two functions, one to initialize the dragons and another one to update them.
function initDragons() {In the function initDragons() , we start with the loop for (i; i<dragons; i++), usually this sort of loops look the same, with a variable to initialize the loop, the condition to loop and the incrementation of the variable value after each loop iteration.
for (i; i<dragons; i++) {
attachMovie("dragon", "dragon"+i, i);
dragon = _root["dragon"+i];
updateDragons(dragon);
dragon.onEnterFrame = function() {
if (this.hitTest(arrows)) {
score += 5;
trace(score);
arrowActive = false;
removeMovieClip(arrows);
updateDragons(this);
}
if (this._x>0) {
this._x -= this.velo;
} else {
updateDragons(this);
}
};
}
}
initDragons();
function updateDragons(which) {
which.gotoAndStop(random(4));
which._x = random(100)+530;
which._y = random(80)+20;
which.velo = random(10)+2;
}
when the value of i is less than the number of dragons, we will attach an instance of our movieclip dragon with this script:
attachMovie("dragon", "dragon"+i, i)
The new name of this instance is "dragon"+i, the value of i is incrementing, so the first one will be called dragon0, the second dragon1 and because we set dragons number as 3, the last instance will be dragon2 finishing the loop.
To make our life easier, we will set a variable to store the name of each dragon, dragon = _root["dragon"+i];
To update the dragons that have been just created, we need to call the function updateDragons(dragon) explained later on.
dragon.onEnterFrame = function() on real time, each intance of the dragons will:
if (this.hitTest(arrows)) check if the arrows hit them, and if it happens,
score += 5; the score will be increased by 5
trace(score); the output window will show us the score
arrowActive = false; the arrow is not active
removeMovieClip(arrows); the arrow will be removed
updateDragons(this); we will update the dragons intance
if (this._x>0) {
this._x -= this.velo;
} else {
updateDragons(this);
}
if the dragon is on the stage, its x position is bigger than zero, it will move horizontally (x axis), if not (when reaches the end of the stage) the dragon will be updated.
initDragons(); we call the function that just declared.
We declare the function to update the dragons, function updateDragons(which)
The x position of the dragon will be randomly chosen, which._x = random(100)+530;
the same with the y position, which._y = random(80)+20;
and the speed the dragon will have, is chosen randomly aswell, which.velo = random(10)+2;
random(number) means that flash will take randomly a number between zero and the number in the brackets, if you want this number to be bigger than zero, then add another number, i.e. random(80)+20 is a number between 20 and 100.
Done! we have a Vertical Shooter game!
updating the script we have:
//---- variables ----Adding a couple of things, you can have something similar to this.
var steps:Number = 5;
var spriteX:Number = 265;
var spriteY:Number = 265;
var speed:Number = 25;
var arrowActive:Boolean = false;
var dragons:Number = 3;
var i:Number = 0;
var score:Number = 0;
//---- properties ----
knight.swapDepths(10);
//---- functions ----
function checkKeys() {
if (Key.isDown(Key.RIGHT) && spriteX<510) {
spriteX += steps;
knight.legs.play();
} else if (Key.isDown(Key.LEFT) && spriteX>40) {
spriteX -= steps;
knight.legs.play();
}
if (Key.isDown(Key.UP) && arrowActive == false) {
knight.arms.play();
attachMovie("arrow", "arrows", 8);
arrows._x = spriteX;
arrows._y = spriteY+50;
//arrowActive = true
}
}
function updatePawel() {
knight._x = spriteX;
knight._y = spriteY;
}
function updateArrow() {
if (arrowActive == true) {
arrows._y -= speed;
}
if (arrows._y<-10) {
arrowActive = false;
removeMovieClip(arrows);
}
}
function initDragons() {
for (i; i<dragons; i++) {
attachMovie("dragon", "dragon"+i, i);
dragon = _root["dragon"+i];
updateDragons(dragon);
dragon.onEnterFrame = function() {
if (this.hitTest(arrows)) {
score += 5;
trace(score);
arrowActive = false;
removeMovieClip(arrows);
updateDragons(this);
}
if (this._x>0) {
this._x -= this.velo;
} else {
updateDragons(this);
}
};
}
}
initDragons();
function updateDragons(which) {
which._x = random(100)+530;
which._y = random(80)+20;
which.velo = random(10)+2;
}
this.onEnterFrame = function() {
checkKeys();
updatePawel();
updateArrow();
};
No .fla as is better for you to learn if you read the tutorial and practice everything yourself.
If any questions, don't hesitate to contact me.
Salut!
No comments:
Post a Comment