A fixed shooter has players only able to control their two-dimensional position on the screen and sometimes the direction they are facing*
*Wikipedia
So, we will create a game using our super hero Pawel to kill dragons that are coming from one side of the screen.
Let's start.
- We need a sprite to make it walk, in this case I use a movieclip of Pawel and as he is a knight, the instance name is knight
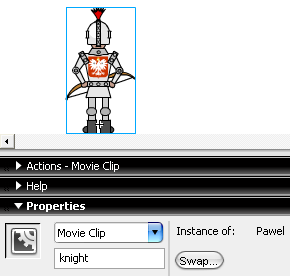
On another layer, in the first frame of our timeline we need the following actionscript:
//---- variables ----Ok, our hero is moving from side to side on the screen but, can still move even outside of the stage!
var steps:Number = 5;
var spriteX:Number = 265;
var spriteY:Number = 265;
//---- functions ----
function checkKeys() {
if (Key.isDown(Key.RIGHT)) {
spriteX += steps;
} else if (Key.isDown(Key.LEFT)) {
spriteX -= steps;
}
}
function updatePawel() {
knight._x = spriteX;
knight._y = spriteY;
}
this.onEnterFrame = function() {
checkKeys();
updatePawel();
};
we can animate the legs and the arms so it will look better and it will add the option of throwing arrows (we don't have arrows yet).
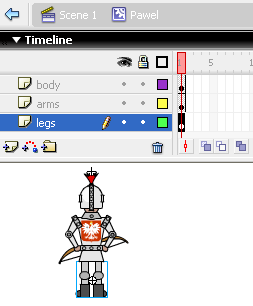
If we edit our movieclip, should have 3 layers; The arms and legs will be movieclips (inside of our movieclip Pawel) and will have their respective instance names.
So, let's update our script (first frame of the main timeline):
//---- variables ----
var steps:Number = 5;
var spriteX:Number = 265;
var spriteY:Number = 265;
//---- functions ----
function checkKeys() {
if (Key.isDown(Key.RIGHT) && spriteX<510) {
spriteX += steps;
knight.legs.play();
} else if (Key.isDown(Key.LEFT) && spriteX>40) {
spriteX -= steps;
knight.legs.play();
}
if (Key.isDown(Key.UP)) {
knight.arms.play();
}
}
function updatePawel() {
knight._x = spriteX;
knight._y = spriteY;
}
this.onEnterFrame = function() {
checkKeys();
updatePawel();
};
First we declare the variables, steps are the number of pixels our hero will move, spriteX and spriteY will help us to give value to the X and Y position of our hero.
The first function checks if we are pressing the keys that are making our hero to do things;
if (Key.isDown(Key.RIGHT) && spriteX<510){
spriteX += steps;
knight.legs.play();
}
means that only if we press the Right arrowKey and our hero is not outside of the stage, our hero will move to the right (remember, steps = 5 pixels) and the legs animation will play.
else if (Key.isDown(Key.LEFT) && spriteX>40) {
spriteX -= steps;
knight.legs.play();
}
same as above but in this case the conditional is if the Left arrowKey of our keyboard is pressed and the hero is not outside of the stage, the hero will move to the left by 5 pixels and the legs movieclip will play.
if (Key.isDown(Key.UP)) {
knight.arms.play();
}
If we press the Up arrowKey on our keyboard, the animation in our arms movieclip will play.
function updatePawel() {
knight._x = spriteX;
knight._y = spriteY;
}
This function is to telling flash that our hero will take the X and Y values of the variables spriteX and spriteY to change our heros position.
this.onEnterFrame = function() {
checkKeys();
updatePawel();
};
On real time, flash will be checking if we are pressing the keys or if our hero is moving.
Check the swf here
Next part of this "tutorial" coming soon
salut!
5 comments:
What was your stage size?
Hi there,
I used the default stage size, ie 550x400 pixels.
Sorry about the problem with the keys, I fixed the code, had a -= steps; as the RIGHT key function, so whether you pressed Left or Right Pawel always went left... but now is sorted as it is += steps as shown in the code above.
Salut
Hello again!
Thank you for responding so quickly to my question. Sorry to bother you yet again, but I just have one more question. I keep receiving the following error message when working in Flash 8. Is this line of code not compatible with this version of Flash?
**Error** Scene=Scene 1, layer=actions, frame=1:Line 11: Unexpected '>' encountered
if (Key.isDown(Key.RIGHT) && spriteX<510)>40) {
I promise I won't bother you again after this and FYI, your written instructions were superb!
Yasou!
hey Yasou, no worries, you are not bothering anyone ;)
well, when I copied this post to send it to Kirupa, blogger mixed up everything as it though the code was XML somehow...
so the code on Kirupa is not correct, try with the code on this page, or on the line that has the:
if (Key.isDown(Key.RIGHT) && spriteX<510)>40) {
take out the )>40 leaving only if (Key.isDown(Key.RIGHT) && spriteX<510){ instead.
Hope it helps.
If anything, Im here
Good luck
hey ernesto... im creating a game based on your tutorial, really grear, but maybe you can help me with the dragons ... they go from right to left... what if i want to have them come from the top.
alex
parece como ke hablaras espaniol... al menos el blog lo hablo :)
Post a Comment